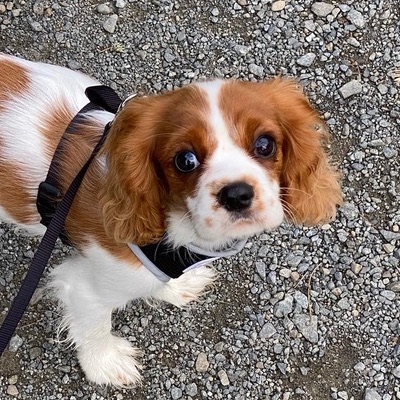
HTML, CSS, & JavaScript
Meet Luna. Luna is a dog. Dogs have a structure and elements, like an
HTML page. The top of the model is dog
, an object which
represents the whole dog
. Beneath the
dog
object are nodes and each of these is another object:
e.g. ears
with the attributes “big", "floppy", and
"fluffy”.
Much like a CSS style sheet King Charles Cavalier Spaniels have
certain specified styles. Luna has a coat color
of
“Blenheim”, an adult height
of 30-33 cm, and her coat is
silky with moderate length
. Among many more features to
her presentation.
Luna has behaviours which operate like JavaScript instructions
providing interactivity, responses to events, and controlling how she
interacts with people, other dogs, and so on. These built-in scripts
include Spaniel behaviours, which can be triggered like a JavaScript
instructions, leading Luna to start hunting()
or
pointing()
: freezing on the spot, with one paw up, and
nose toward something she wants to bring to our attention.
Control flow in JavaScript
Control flow in JavaScript is how your computer runs code from top to
bottom. It starts from the first line and ends at the last line,
unless it hits any statement that changes the control flow of the
program such as loops, conditionals, or functions. Luna may start her
day by waking up from sleep()
and going to
eatBreakfast()
. After she has finished
eatBreakfast()
she may want to play fetch()
.
Loops in JavaScript
Luna may enter a loop at times, especially when she wants to play
fetch()
. Based on the condition of Luna (and her pet
parents) wanting to play fetch throwBall()
is called,
until either Luna or the pet parent is tired of playing
fetch()
. There could be events throughout the day, and
Luna may start eat()
, warn()
,
hunting()
, pointing()
, or simply
sleep()
.
The pet parents will have to abide by Luna until the loop has finished.
The Document Object Model
The API of the browser, the Document Object Model (DOM) is quite awful, and JavaScript is unfairly blamed. The DOM would be painful to work with in any language. The DOM is poorly specified and inconsistently implemented.
Excerpt from JavaScript: The Good Parts by Douglas Crockford.
The Document Object Model (‘DOM’) is a data representation of the objects that comprise the structure and content of a document on the web. The DOM is a programming interface that represents the page so that programs can change the document structure, style, and content.
The page is received as HTML code sent by the web server. At the top of the model is the document object, which represents the whole document (the web page). Beneath the document object each box is called a node, which is another object.
The DOM is a web API designed for the web browser to build websites
using their rendering and scripting engines. It can be accessed using
JavaScript, but the DOM is accessible independent of any particular
programming language. You can immediately start accessing the DOM
either through an inline <script>
element or by
linking a script to the web page.
This could be as simple as a mildly annoying pop-up welcoming users to your page:
<body onload="alert('Welcome to my home page!');">
Accessing data - arrays vs objects
Arrays
The array is a list-like object for storing data. It allows us to store multiple values under a single variable name. We can efficiently loop through an array and access each value individually, doing the same thing to every value. This is a more efficient storage method for data than the alternative of storing every value in its own variable. Arrays consist of square brackets and items separated by commas.
Suppose that Luna wants to keep track of all of her toys:
const
toys = ['Kevin the Duck', 'Ranger',
'Yummy Rope', 'Tennis Ball', 'Orange Rubber Ball', 'Towel'];
To access a value from the array we simply use the items index, starting from 0. So:
toys[0]
='Kevin the Duck'
toys[3]
='Tennis Ball'
toys[5]
='Towel'
Objects
As you may have noticed by now most things are objects, including core JavaScript features such as arrays and the DOM. We can create our own objects as a collection of related data and/or functionality.
We could use an object to store Luna’s characteristics:
const
dog = {
name: 'Luna',
age: '5 months',
breed: 'King Charles
Cavalier Spaniel',
coatColour: 'Blenheim',
favouriteToy: 'Kevin
the Duck'
};
To access a value in this object we use dot notation, the syntax looks
like this: object.property
.
For example:
dog.name
='Luna'
dog.age
='5 months'
dog.breed
='King Charles Cavalier Spaniel'
dog.coatColour
='Blenheim'
dog.favouriteToy
='Kevin the Duck'
The usefulness of functions
Generally programming is about factoring a task into a set of functions and data structures. Functions let us store a single task in a block of code, which we can use with a short command – rather than having to re-enter the same code or manually repeat the same task multiple times.
A function in JavaScript looks like this:
function myFunction() {
// code goes
here
}
Here's how we can use the function:
myFunction();
We may want to have a function that takes a parameter:
function myFunction(input) {
alert(input)
}
Which we will call and pass a parameter to:
myFunction('Hello World!');
We can also pass multiple parameters to a function:
function myFunction(input1, input2) {
alert(input1
+ input2)
}
Which we will call and pass two parameters to:
myFunction('Hello ', 'World!');
Functions are useful for re-using code and for keeping our code DRY.